双目相机标定
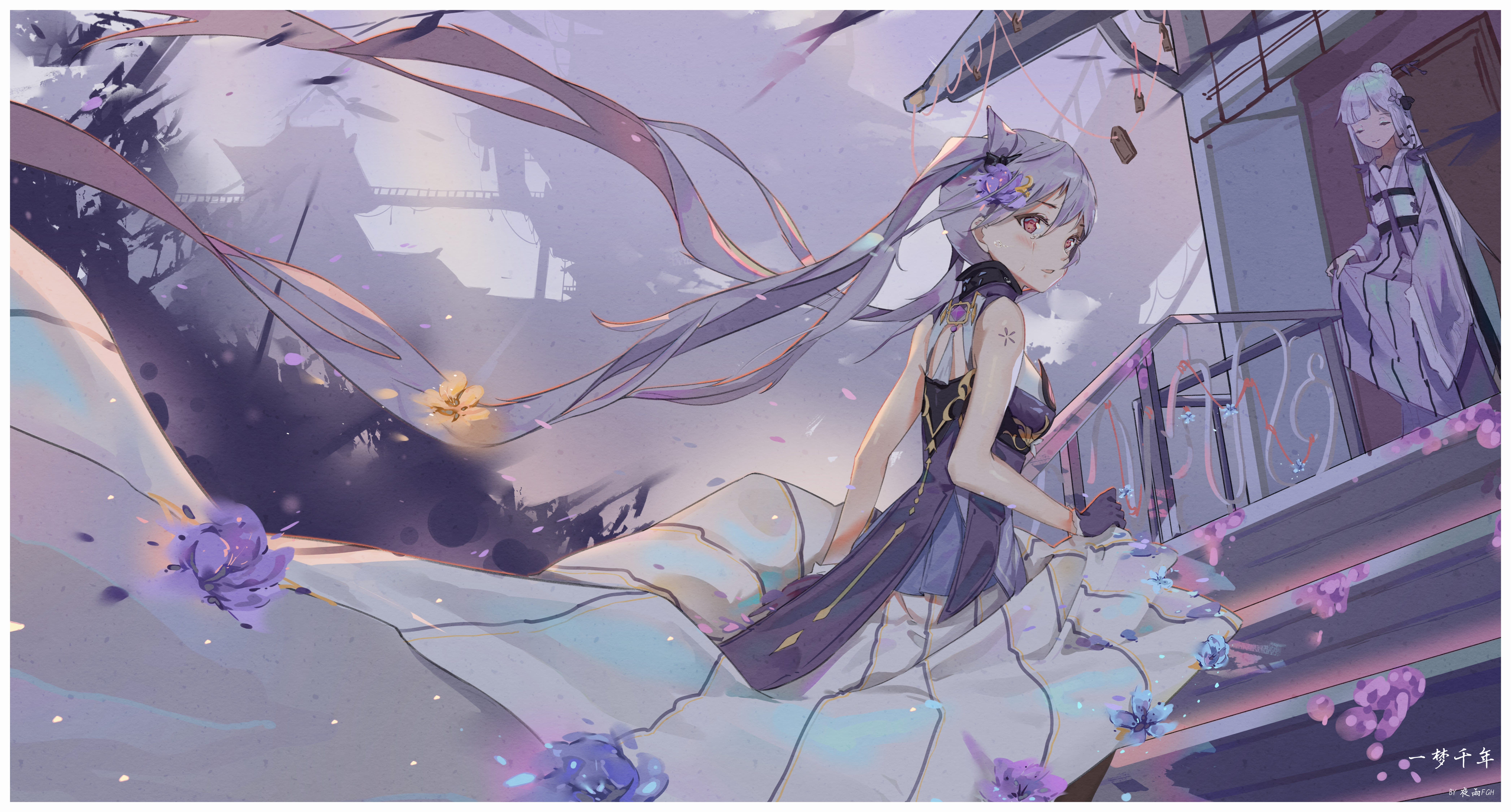
双目相机标定
张凯博前言
相机标定是计算机视觉的基础。由于物理原因,相机采集到的图像存在一定的畸变需要进行标定,从而矫正图像。为后期计算视差图、识别等做准备。
生成标定板
在标定前需要标定板帮助我们进行标定。对于一般标定,无需昂贵的高精度标定板,下面推荐一个生成标定板的网站。
相机校正
相机校正包括单目校正和双目校正两个步骤,其中单目校正主要计算出相机的内参,来对镜头进行去畸变以及深度的推算。双目校正则是计算出左右相机的外参,知道外参后可以将左右相机分别旋转一定角度,以至左右相机的同名点在同一平面且同一水平线上。
实验
本实验完整代码已上传到Github
中,下面内容为实验过程及代码说明。
实验器材
本次使用双目相机进行试验。此双目相机由一颗可见光相机和一颗红外相机组成。
对于三维重建,使用双彩色相机进行图像采集更为合适。此款相机更适合对于复杂环境或特殊用途,如活体人脸识别等。但对于此双目相机标定试验仍可以进行试验。
环境搭建
本实验使用OpenCV进行,需要进行相应的环境搭建。
使用Anaconda
创建解释器环境。并使用pip进行安装。
1 | pip install opencv-python |
拍摄左右相机图片
使用双目相机同时拍摄标定板,并将图像存放到对应的文件夹中,并进行编号。
新建名为左右相机拍摄.py
的文件,在文件中添加如下代码。
1 |
|
双目摄像头矫正
获取角点
从拍摄的棋盘图像中提取角点。
在获取角点时,我们使用cv2.findChessboardCorners
方法来检测角点,并使用cv2.cornerSubPix
方法提高角点的精度。
chessboard_size = (9, 14)
为棋盘内角点的数量,请根据实际棋盘大小进行修改。
1 | import cv2 |
进行单目标定
通过提取到的角点,使用cv2.calibrateCamera
对左右相机分别进行标定,得到每个相机的内参(相机矩阵),畸变系数,旋转向量和平移向量。
内参(Camera Matrix)
:每个相机都有一个 3x3 的内参矩阵(mtx_l 和 mtx_r)。
畸变系数(Distortion Coefficients)
:每个相机的畸变参数(dist_l 和 dist_r),通常包含 5 个参数。
旋转向量和平移向量
:描述相机坐标系与世界坐标系之间的关系。
1 | # 校准左相机 |
进行双目标定
使用左右相机拍摄的棋盘图像,计算出两相机之间的旋转和平移关系。
旋转矩阵 (R)
:描述两个相机之间的旋转关系。
平移向量 (T)
:描述两个相机之间的平移关系。
本质矩阵 (E)
:两个相机的内参矩阵已知时,给出两相机坐标系之间的关系。
基础矩阵 (F)
:计算两个相机图像平面之间的关系。
1 | # 立体校正 |
立体校正
在双目标定后,我们可以进行立体校正(Stereo Rectification),将左右相机的图像映射到相同的平面,方便后续的视差计算。
1 | # 计算重映射矩阵 |
矫正图像
使用等线来观察矫正效果。
1 | def stack_images(img1, img2): |
完整代码
1 | import cv2 |
实验结果分析
左右摄像头画面的区别
可以从图中看出,由于两摄像头的物理位置原因,同时拍摄的物体在不同摄像头的画面的左右摄像头画面存在差异,左摄像头可以拍摄完整的图像,而右侧摄像头拍摄的棋盘不是完整的。
左右相机棋盘图像数据
使用相机拍摄程序,同时使用左右相机对棋盘进行不同角度的拍摄,在对应的文件夹中图片的数量是一致的,且同一时刻的图像使用相同的编号。
角点检测
分别对左右相机拍摄的棋盘图像进行角点检测,利用检测到的角点,进行校正参数的计算。
计算校正映射
校正参数的计算结果如下
1 | 输出校正参数 |
矫正前后对比
使用计算得到的矫正参数,将左右相机拍摄的棋盘图像进行校正,并显示对比。
矫正前后的对比图如下
分析对比图我们可以很明显的观察到,矫正前后的图像是不同的。不同的位置如图所示。
左相机经过校正后,在图像的右侧出现的空白区域,证明了整体画面是经过调整过的。
右相机经过校正后,在图像的上侧出现的空白区域,且左下角区域的位置出现了调整,说明对画面的位置进行了有效的矫正。
等线分析
绘制等线。观察左右相机拍摄同一物体,对应的像素是否在同一水平线上。
在左右相机合成图像上,观察等线与像素点,可以很明显的观察到同一物体对应的像素点都在同一水平线上。
总结
由于本实验使用的是双目相机,在物理上已经尽可能的保持了两个相机的间距是固定且是水平的,但由于安装等原因,避免不了的存在一定的误差。经过标定和矫正进一步的使得图像的误差变得非常小。
但本次使用的双目相机是红外与彩色相机,彩色相机与红外相机的图像可能在纹理、亮度、对比度等方面差异较大,对于特征点的提取存在一定的困难。若后期进行测距、视差、三维重建等工作,双彩色相机是更为合适的。
本实验,是基于张氏标定法进行的标定,此方法存在一定的弊端。
-
此方法严重依赖于标定板,标定板若出现损坏、不完整、变形等情况,会造成标定参数的误差。
-
需要多角度拍摄标定板,若对于某些设备无法完成多角度的拍摄,则会大大降低矫正效果。
-
对于需要高精度的场景,则需要高精度的标定板,高精度标定的生产难度较大导致价格较昂贵。
-
在拍摄标定板时,不能出现移动的情况,否则可能会造成标定参数的误差。
在相机和镜头的选择上,尽可能的使用固定间距的双目相机,且使用无畸变镜头,虽然矫正算法可能对画面进行一定程度的矫正,但是对于畸变严重的情况,会出现大面积裁切的情况,无法尽可能的保留有效图像信息。